Reading and Writing Mapdl Archive Files#
Reading ANSYS Archives#
MAPDL archive *.cdb
and *.dat
files containing elements (both
legacy and modern) can be loaded using Archive and then converted to a
vtk
object:
from ansys.mapdl import reader as pymapdl_reader
from ansys.mapdl.reader import examples
# Read a sample archive file
archive = pymapdl_reader.Archive(examples.hexarchivefile)
# Print various raw data from cdb
print(archive.nnum, archive.nodes)
# access a vtk unstructured grid from the raw data and plot it
grid = archive.grid
archive.plot(color='w', show_edges=True)
You can also optionally read in any stored parameters within the
archive file by enabling the read_parameters
parameter.
from ansys.mapdl import reader as pymapdl_reader
archive = pymapdl_reader.Archive('mesh.cdb', read_parameters=True)
# parameters are stored as a dictionary
archive.parameters
See the Archive class documentation below for more details on the class methods and properties.
Writing ANSYS Archives#
Unstructured grids generated using VTK can be converted to ANSYS APDL
archive files and loaded into any version of ANSYS using
pymapdl_reader.save_as_archive
. The following example using the
built-in archive file demonstrates this capability.
import pyvista as pv
from pyvista import examples
from ansys.mapdl import reader as pymapdl_reader
# load in a vtk unstructured grid
grid = pv.UnstructuredGrid(examples.hexbeamfile)
script_filename = '/tmp/grid.cdb'
pymapdl_reader.save_as_archive(script_filename, grid)
# optionally read in archive in ANSYS and generate cell shape
# quality report
from ansys.mapdl.core import launch_mapdl
mapdl = launch_mapdl()
mapdl.cdread('db', script_filename)
mapdl.prep7()
mapdl.shpp('SUMM')
Resulting ANSYS quality report:
------------------------------------------------------------------------------
<<<<<< SHAPE TESTING SUMMARY >>>>>>
<<<<<< FOR ALL SELECTED ELEMENTS >>>>>>
------------------------------------------------------------------------------
--------------------------------------
| Element count 40 SOLID185 |
--------------------------------------
Test Number tested Warning count Error count Warn+Err %
---- ------------- ------------- ----------- ----------
Aspect Ratio 40 0 0 0.00 %
Parallel Deviation 40 0 0 0.00 %
Maximum Angle 40 0 0 0.00 %
Jacobian Ratio 40 0 0 0.00 %
Warping Factor 40 0 0 0.00 %
Any 40 0 0 0.00 %
------------------------------------------------------------------------------
Converting a MAPDL Archive File to VTK for Paraview#
MAPDL archive files containing solid elements (both legacy and modern) can be loaded using Archive and then converted to a VTK object.
from ansys.mapdl import reader as pymapdl_reader
from ansys.mapdl.reader import examples
# Sample *.cdb
filename = examples.hexarchivefile
# Read ansys archive file
archive = pymapdl_reader.Archive(filename)
# Print overview of data read from cdb
print(archive)
# Create a vtk unstructured grid from the raw data and plot it
grid = archive.parse_vtk(force_linear=True)
grid.plot(color='w', show_edges=True)
# save this as a vtk xml file
grid.save('hex.vtu')
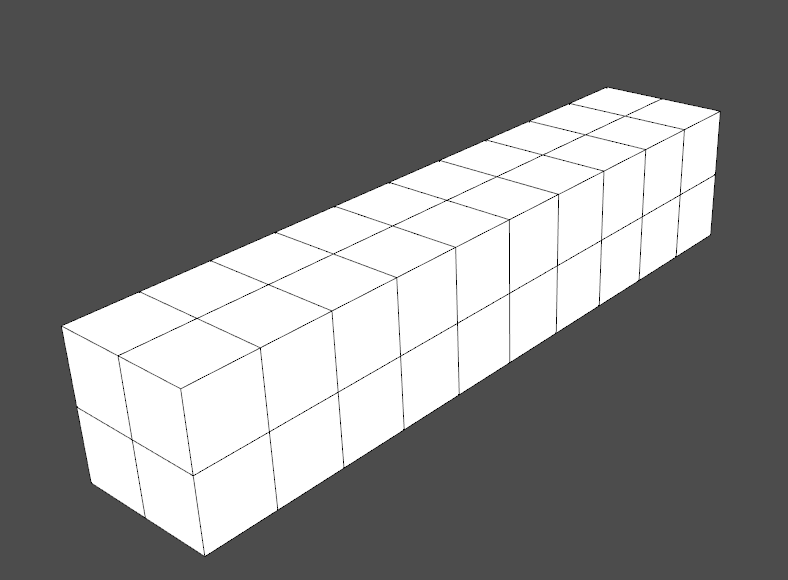
You can then load this vtk file using pyvista
or another program that uses VTK.
# Load this from vtk
import pyvista as pv
grid = pv.read('hex.vtk')
grid.plot()
Supported Elements#
At the moment, only solid elements are supported by the
save_as_archive
function, to include:
vtk.VTK_TETRA
vtk.VTK_QUADRATIC_TETRA
vtk.VTK_PYRAMID
vtk.VTK_QUADRATIC_PYRAMID
vtk.VTK_WEDGE
vtk.VTK_QUADRATIC_WEDGE
vtk.VTK_HEXAHEDRON
vtk.VTK_QUADRATIC_HEXAHEDRON
Linear element types will be written as SOLID185, quadratic elements will be written as SOLID186, except for quadratic tetrahedrals, which will be written as SOLID187.
Archive Class#
- class ansys.mapdl.reader.archive.Archive(filename, read_parameters=False, parse_vtk=True, force_linear=False, allowable_types=None, null_unallowed=False, verbose=False, name='', read_eblock=True)#
Read a blocked ANSYS archive file or input file.
Reads a blocked CDB file and optionally parses it to a vtk grid. This can be used to read in files written from MAPDL using the
CDWRITE
command or input files ('.dat'
) files written from ANSYS Workbench.Write the archive file using
CDWRITE, DB, archive.cdb
- Parameters:
filename (string, pathlib.Path) – Filename of block formatted cdb file
read_parameters (bool, optional) – Optionally read parameters from the archive file. Default
False
.parse_vtk (bool, optional) – When
True
, parse the raw data into to VTK format.force_linear (bool, optional) – This parser creates quadratic elements if available. Set this to True to always create linear elements. Defaults to False.
allowable_types (list, optional) –
Allowable element types. Defaults to all valid element types in
ansys.mapdl.reader.elements.valid_types
See
help(ansys.mapdl.reader.elements)
for available element types.null_unallowed (bool, optional) – Elements types not matching element types will be stored as empty (null) elements. Useful for debug or tracking element numbers. Default False.
verbose (bool, optional) – Print out each step when reading the archive file. Used for debug purposes and defaults to
False
.name (str, optional) – Internally used parameter used to have a custom
__repr__
.read_eblock (bool, default: True) – Read the element block.
Examples
>>> from ansys.mapdl import reader as pymapdl_reader >>> from ansys.mapdl.reader import examples >>> hex_beam = pymapdl_reader.Archive(examples.hexarchivefile) >>> print(hex_beam) ANSYS Archive File HexBeam.cdb Number of Nodes: 40 Number of Elements: 321 Number of Element Types: 1 Number of Node Components: 2 Number of Element Components: 2
Print the node array
>>> hex_beam.nodes array([[0. , 0. , 0. ], [1. , 0. , 0. ], [0.25, 0. , 0. ], ..., [0.75, 0.5 , 3.5 ], [0.75, 0.5 , 4. ], [0.75, 0.5 , 4.5 ]])
Read an ANSYS workbench input file
>>> my_archive = pymapdl_reader.Archive('C:\Users\user\stuff.dat')
Notes
This class only reads EBLOCK records with SOLID records. For example, the record
EBLOCK,19,SOLID,,3588
will be read, butEBLOCK,10,,,3588
will not be read. Generally, MAPDL will only write SOLID records and Mechanical Workbench may write SOLID records. These additional records will be ignored.- property ekey#
Element type key
Array containing element type numbers in the first column and the element types (like SURF154) in the second column.
Examples
>>> from ansys.mapdl import reader as pymapdl_reader >>> from ansys.mapdl.reader import examples >>> archive = pymapdl_reader.Archive(examples.hexarchivefile) >>> archive.ekey array([[ 1, 45], [ 2, 95], [ 3, 92], [ 60, 154]], dtype=int32)
- property elem#
List of elements containing raw ansys information.
Each element contains 10 items plus the nodes belonging to the element. The first 10 items are:
FIELD 0 : material reference number
FIELD 1 : element type number
FIELD 2 : real constant reference number
FIELD 3 : section number
FIELD 4 : element coordinate system
FIELD 5 : death flag (0 - alive, 1 - dead)
FIELD 6 : solid model reference
FIELD 7 : coded shape key
FIELD 8 : element number
FIELD 9 : base element number (applicable to reinforcing elements only)
FIELDS 10 - 30 : The nodes belonging to the element in ANSYS numbering.
Examples
>>> from ansys.mapdl import reader as pymapdl_reader >>> from ansys.mapdl.reader import examples >>> archive = pymapdl_reader.Archive(examples.hexarchivefile) >>> archive.elem [array([ 1, 4, 19, 15, 63, 91, 286, 240, 3, 18, 17, 16, 81, 276, 267, 258, 62, 90, 285, 239], array([ 4, 2, 8, 19, 91, 44, 147, 286, 5, 7, 21, 18, 109, 137, 313, 276, 90, 43, 146, 285], array([ 15, 19, 12, 10, 240, 286, 203, 175, 17, 20, 13, 14, 267, 304, 221, 230, 239, 285, 202, 174], ...
- property elem_real_constant#
Real constant reference for each element.
Use the data within
rlblock
andrlblock_num
to get the real constant datat for each element.Examples
>>> from ansys.mapdl import reader as pymapdl_reader >>> from ansys.mapdl.reader import examples >>> archive = pymapdl_reader.Archive(examples.hexarchivefile) >>> archive.elem_real_constant array([ 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, ..., 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 61, 61, 61, 61, 61, 61, 61, 61, 61, 61, 61, 61, 61, 61, 61, 61, 61, 61, 61], dtype=int32)
- property element_components#
Element components for the archive.
Output is a dictionary of element components. Each entry is an array of MAPDL element numbers corresponding to the element component. The keys are element component names.
Examples
>>> from ansys.mapdl import reader as pymapdl_reader >>> from ansys.mapdl.reader import examples >>> archive = pymapdl_reader.Archive(examples.hexarchivefile) >>> archive.element_components {'ECOMP1 ': array([17, 18, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32, 33, 34, 35, 36, 37, 38, 39, 40], dtype=int32), 'ECOMP2 ': array([ 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 23, 24], dtype=int32)}
- element_coord_system()#
Element coordinate system number
Examples
>>> from ansys.mapdl import reader as pymapdl_reader >>> from ansys.mapdl.reader import examples >>> archive = pymapdl_reader.Archive(examples.hexarchivefile) >>> archive.element_coord_system array([0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0], dtype=int32)
- property enum#
ANSYS element numbers.
Examples
>>> from ansys.mapdl import reader as pymapdl_reader >>> from ansys.mapdl.reader import examples >>> archive = pymapdl_reader.Archive(examples.hexarchivefile) >>> archive.enum array([ 1, 2, 3, ..., 9998, 9999, 10000])
- property et_id#
Element type id (ET) for each element.
- property etype#
Element type of each element.
This is the ansys element type for each element.
Examples
>>> from ansys.mapdl import reader as pymapdl_reader >>> from ansys.mapdl.reader import examples >>> archive = pymapdl_reader.Archive(examples.hexarchivefile) >>> archive.etype array([ 45, 45, 45, 45, 45, 45, 45, 45, 45, 45, 45, 45, 45, 45, 45, 45, 45, 45, 45, 92, 92, 92, 92, 92, 92, 92, 92, 92, 92, 92, 92, 92, 92, ..., 92, 92, 92, 92, 92, 154, 154, 154, 154, 154, 154, 154, 154, 154, 154, 154, 154, 154, 154, 154, 154, 154, 154], dtype=int32)
Notes
Element types are listed below. Please see the APDL Element Reference for more details:
https://www.mm.bme.hu/~gyebro/files/vem/ansys_14_element_reference.pdf
- property grid#
Return a
pyvista.UnstructuredGrid
of the archive file.Examples
>>> from ansys.mapdl import reader as pymapdl_reader >>> from ansys.mapdl.reader import examples >>> archive = pymapdl_reader.Archive(examples.hexarchivefile) >>> archive.grid UnstructuredGrid (0x7ffa237f08a0) N Cells: 40 N Points: 321 X Bounds: 0.000e+00, 1.000e+00 Y Bounds: 0.000e+00, 1.000e+00 Z Bounds: 0.000e+00, 5.000e+00 N Arrays: 13
- property key_option#
Additional key options for element types
Examples
>>> from ansys.mapdl import reader as pymapdl_reader >>> from ansys.mapdl.reader import examples >>> archive = pymapdl_reader.Archive(examples.hexarchivefile) >>> archive.key_option {1: [[1, 11]]}
- property material_type#
Material type index of each element in the archive.
Examples
>>> from ansys.mapdl import reader as pymapdl_reader >>> from ansys.mapdl.reader import examples >>> archive = pymapdl_reader.Archive(examples.hexarchivefile) >>> archive.material_type array([1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1], dtype=int32)
- property n_elem#
Number of nodes
- property n_node#
Number of nodes
- property nnum#
Array of node numbers.
Examples
>>> from ansys.mapdl import reader as pymapdl_reader >>> from ansys.mapdl.reader import examples >>> archive = pymapdl_reader.Archive(examples.hexarchivefile) >>> archive.nnum array([ 1, 2, 3, ..., 19998, 19999, 20000])
- property node_angles#
Node angles from the archive file.
Examples
>>> from ansys.mapdl import reader as pymapdl_reader >>> from ansys.mapdl.reader import examples >>> archive = pymapdl_reader.Archive(examples.hexarchivefile) >>> archive.nodes [[0. 0. 0. ] [0. 0. 0. ] [0. 0. 0. ] ..., [0. 0. 0. ] [0. 0. 0. ] [0. 0. 0. ]]
- property node_components#
Node components for the archive.
Output is a dictionary of node components. Each entry is an array of MAPDL node numbers corresponding to the node component. The keys are node component names.
Examples
>>> from ansys.mapdl import reader as pymapdl_reader >>> from ansys.mapdl.reader import examples >>> archive = pymapdl_reader.Archive(examples.hexarchivefile) >>> archive.node_components {'NCOMP2 ': array([ 1, 2, 3, 4, 5, 6, 7, 8, 14, 15, 16, 17, 18, 19, 20, 21, 43, 44, 62, 63, 64, 81, 82, 90, 91, 92, 93, 94, 118, 119, 120, 121, 122, 123, 124, 125, 126, 137, 147, 148, 149, 150, 151, 152, 153, 165, 166, 167, 193, 194, 195, 202, 203, 204, 205, 206, 207, 221, 240, 258, 267, 268, 276, 277, 278, 285, 286, 287, 304, 305, 306, 313, 314, 315, 316 ], dtype=int32), ..., }
- property nodes#
Array of nodes.
Examples
>>> from ansys.mapdl import reader as pymapdl_reader >>> from ansys.mapdl.reader import examples >>> archive = pymapdl_reader.Archive(examples.hexarchivefile) >>> archive.nodes [[0. 0. 0. ] [1. 0. 0. ] [0.25 0. 0. ] ..., [0.75 0.5 3.5 ] [0.75 0.5 4. ] [0.75 0.5 4.5 ]]
- property parameters#
Parameters stored in the archive file
Examples
>>> from ansys.mapdl import reader as pymapdl_reader >>> from ansys.mapdl.reader import examples >>> archive = pymapdl_reader.Archive(examples.hexarchivefile, read_parameters=True) >>> archive.parameters {}
- property pathlib_filename: Path#
Return the
pathlib.Path
version of the filename. This property can not be set.
- plot(off_screen=None, full_screen=None, screenshot=None, interactive=True, cpos=None, window_size=None, show_bounds=False, show_axes=None, notebook=None, background=None, text='', return_img=False, eye_dome_lighting=False, volume=False, parallel_projection=False, jupyter_backend=None, return_viewer=False, return_cpos=False, jupyter_kwargs=None, theme=None, anti_aliasing=None, zoom=None, border=False, border_color='k', border_width=2.0, ssao=False, **kwargs)#
Plot a PyVista, numpy, or vtk object.
- Parameters:
var_item (pyvista.DataSet) – See
Plotter.add_mesh
for all supported types.off_screen (bool, optional) – Plots off screen when
True
. Helpful for saving screenshots without a window popping up. Defaults to the global settingpyvista.OFF_SCREEN
.full_screen (bool, default:
pyvista.plotting.themes.Theme.full_screen
) – Opens window in full screen. When enabled, ignoreswindow_size
.screenshot (str or bool, optional) –
Saves screenshot to file when enabled. See:
Plotter.screenshot()
. DefaultFalse
.When
True
, takes screenshot and returnsnumpy
array of image.interactive (bool, default:
pyvista.plotting.themes.Theme.interactive
) – Allows user to pan and move figure.cpos (list, optional) – List of camera position, focal point, and view up.
window_size (sequence, default:
pyvista.plotting.themes.Theme.window_size
) – Window size in pixels.show_bounds (bool, default: False) – Shows mesh bounds when
True
.show_axes (bool, default:
pyvista.plotting.themes._AxesConfig.show
) – Shows a vtk axes widget.notebook (bool, default:
pyvista.plotting.themes.Theme.notebook
) – WhenTrue
, the resulting plot is placed inline a jupyter notebook. Assumes a jupyter console is active.background (ColorLike, default:
pyvista.plotting.themes.Theme.background
) – Color of the background.text (str, optional) – Adds text at the bottom of the plot.
return_img (bool, default: False) – Returns numpy array of the last image rendered.
eye_dome_lighting (bool, optional) – Enables eye dome lighting.
volume (bool, default: False) – Use the
Plotter.add_volume()
method for volume rendering.parallel_projection (bool, default: False) – Enable parallel projection.
jupyter_backend (str, default:
pyvista.plotting.themes.Theme.jupyter_backend
) –Jupyter notebook plotting backend to use. One of the following:
'none'
: Do not display in the notebook.'static'
: Display a static figure.'trame'
: Display usingtrame
.
This can also be set globally with
pyvista.set_jupyter_backend()
.return_viewer (bool, default: False) – Return the jupyterlab viewer, scene, or display object when plotting with jupyter notebook.
return_cpos (bool, default: False) – Return the last camera position from the render window when enabled. Defaults to value in theme settings.
jupyter_kwargs (dict, optional) – Keyword arguments for the Jupyter notebook plotting backend.
theme (pyvista.plotting.themes.Theme, optional) – Plot-specific theme.
anti_aliasing (str | bool, default:
pyvista.plotting.themes.Theme.anti_aliasing
) – Enable or disable anti-aliasing. IfTrue
, uses"msaa"
. If False, disables anti_aliasing. If a string, should be either"fxaa"
or"ssaa"
.zoom (float, str, optional) – Camera zoom. Either
'tight'
or a float. A value greater than 1 is a zoom-in, a value less than 1 is a zoom-out. Must be greater than 0.border (bool, default: False) – Draw a border around each render window.
border_color (ColorLike, default: "k") –
Either a string, rgb list, or hex color string. For example:
color='white'
color='w'
color=[1.0, 1.0, 1.0]
color='#FFFFFF'
border_width (float, default: 2.0) – Width of the border in pixels when enabled.
ssao (bool, optional) – Enable surface space ambient occlusion (SSAO). See
Plotter.enable_ssao()
for more details.**kwargs (dict, optional) – See
pyvista.Plotter.add_mesh()
for additional options.
- Returns:
cpos (list) – List of camera position, focal point, and view up. Returned only when
return_cpos=True
or set in the default global or plot theme. Not returned when in a jupyter notebook andreturn_viewer=True
.image (np.ndarray) – Numpy array of the last image when either
return_img=True
orscreenshot=True
is set. Not returned when in a jupyter notebook withreturn_viewer=True
. Optionally contains alpha values. Sized:[Window height x Window width x 3] if the theme sets
transparent_background=False
.[Window height x Window width x 4] if the theme sets
transparent_background=True
.
widget (ipywidgets.Widget) – IPython widget when
return_viewer=True
.
Examples
Plot a simple sphere while showing its edges.
>>> import pyvista as pv >>> mesh = pv.Sphere() >>> mesh.plot(show_edges=True)
Plot a volume mesh. Color by distance from the center of the ImageData. Note
volume=True
is passed.>>> import numpy as np >>> grid = pv.ImageData(dimensions=(32, 32, 32), spacing=(0.5, 0.5, 0.5)) >>> grid['data'] = np.linalg.norm(grid.center - grid.points, axis=1) >>> grid['data'] = np.abs(grid['data'] - grid['data'].max()) ** 3 >>> grid.plot(volume=True)
- property quality#
Minimum scaled jacobian cell quality.
Negative values indicate invalid cells while positive values indicate valid cells. Varies between -1 and 1.
Examples
>>> from ansys.mapdl import reader as pymapdl_reader >>> from ansys.mapdl.reader import examples >>> archive = pymapdl_reader.Archive(examples.hexarchivefile) >>> archive.quality array([1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1.])
- property rlblock#
Real constant data from the RLBLOCK.
Examples
>>> from ansys.mapdl import reader as pymapdl_reader >>> from ansys.mapdl.reader import examples >>> archive = pymapdl_reader.Archive(examples.hexarchivefile) >>> archive.rlblock [[0. , 0. , 0. , 0. , 0. , 0. , 0.02 ], [0. , 0. , 0. , 0. , 0. , 0. , 0.01 ], [0. , 0. , 0. , 0. , 0. , 0. , 0.005], [0. , 0. , 0. , 0. , 0. , 0. , 0.005]]
- property rlblock_num#
Indices from the real constant data
Examples
>>> from ansys.mapdl import reader as pymapdl_reader >>> from ansys.mapdl.reader import examples >>> archive = pymapdl_reader.Archive(examples.hexarchivefile) >>> archive.rnum array([60, 61, 62, 63])
- save(filename, binary=True, force_linear=False, allowable_types=[], null_unallowed=False)#
Save the geometry as a vtk file
- Parameters:
filename (str, pathlib.Path) – Filename of output file. Writer type is inferred from the extension of the filename.
binary (bool, optional) – If
True
, write as binary, else ASCII.force_linear (bool, optional) – This parser creates quadratic elements if available. Set this to True to always create linear elements. Defaults to False.
allowable_types (list, optional) –
Allowable element types. Defaults to all valid element types in
ansys.mapdl.reader.elements.valid_types
See
help(ansys.mapdl.reader.elements)
for available element types.null_unallowed (bool, optional) – Elements types not matching element types will be stored as empty (null) elements. Useful for debug or tracking element numbers. Default False.
Examples
>>> geom.save('mesh.vtk')
Notes
Binary files write much faster than ASCII and have a smaller file size.
- property section#
Section number
Examples
>>> from ansys.mapdl import reader as pymapdl_reader >>> from ansys.mapdl.reader import examples >>> archive = pymapdl_reader.Archive(examples.hexarchivefile) >>> archive.section array([1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1], dtype=int32)
- property tshape#
Tshape of contact elements.
- property tshape_key#
Dict with the mapping between element type and element shape.
TShape is only applicable to contact elements.