Compute Cell Quality#
Compute the minimum scaled jacoabian of a vtk.Unstructured.Grid
,
pyvista.UnstructuredGrid
, or pyvista.StructuredGrid
.
Plot Cell Quality#
This built-in example displays the minimum scaled jacobian of each element of a tetrahedral beam:
from pyansys import examples
examples.show_cell_qual()
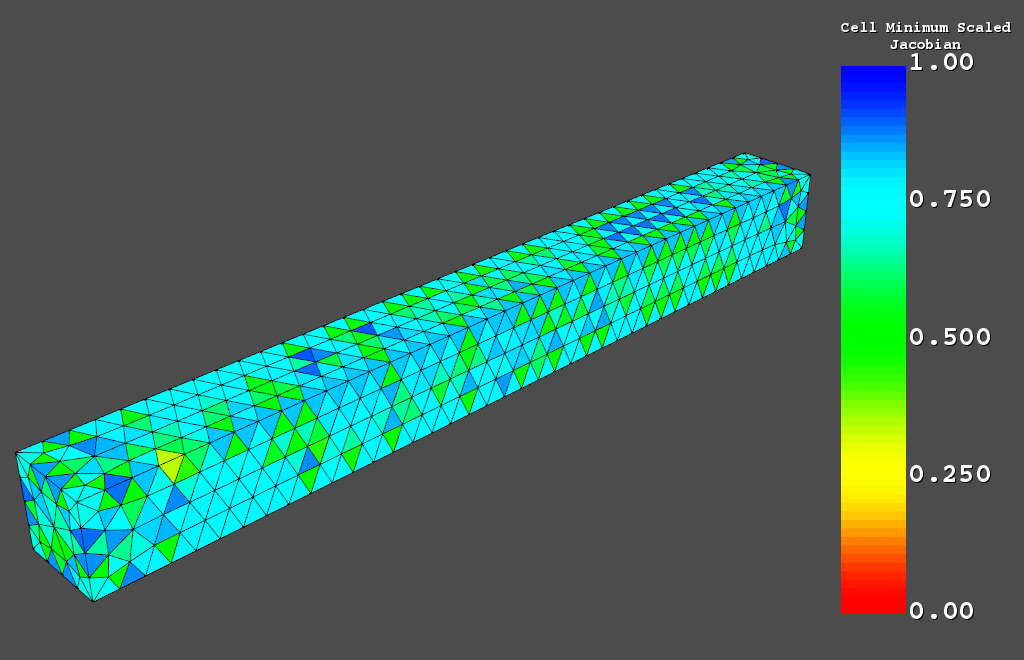
This is the source code for the example:
import pyansys
# load archive file and parse for subsequent FEM queries
from pyansys import examples
# archive = pyansys.Archive(examples.hexarchivefile)
archive = pyansys.Archive(examples.tetarchivefile)
# create vtk object
grid = archive.parse_vtk(force_linear=True)
# get the cell quality
qual = grid.quality
# plot cell quality
grid.plot(scalars=qual, stitle='Cell Minimum Scaled\nJacobian', rng=[0, 1])
pyansys.quality#
- ansys.mapdl.reader.quality(grid)#
Compute the minimum scaled Jacobian cell quality of an UnstructuredGrid.
Negative values indicate invalid cells while positive values indicate valid cells. Varies between -1 and 1.
- Parameters:
grid (pyvista.UnstructuredGrid or pyvista.StructuredGrid) – Structured or Unstructured Grid from
pyvista
.
Examples
>>> from ansys.mapdl import reader as pymapdl_reader >>> import pyvista as pv >>> x = np.arange(-10, 10, 5) >>> y = np.arange(-10, 10, 5) >>> z = np.arange(-10, 10, 5) >>> x, y, z = np.meshgrid(x, y, z) >>> grid = pv.StructuredGrid(x, y, z) >>> pymapdl_reader.quality(grid) array([1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1.])